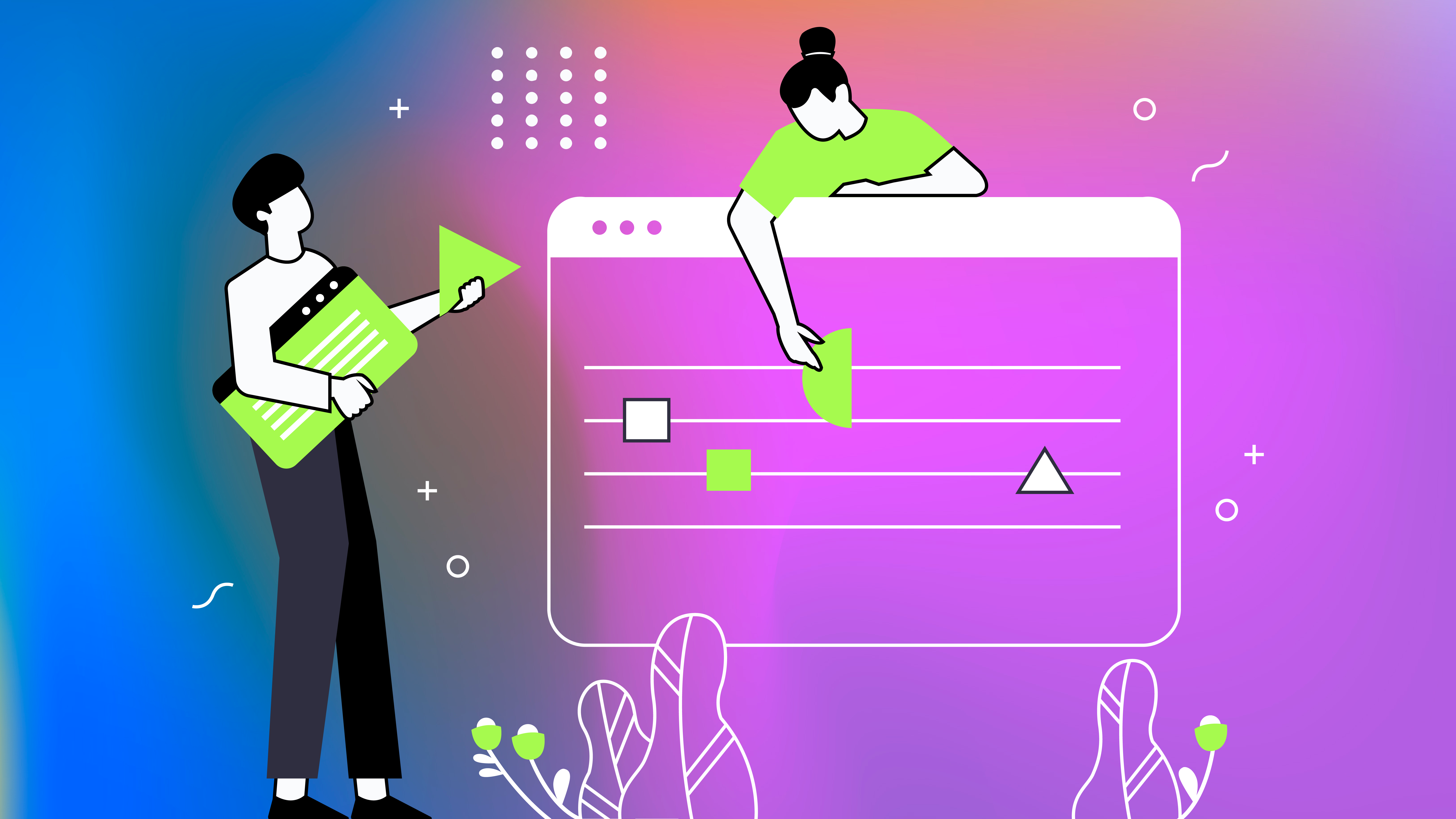
String is a primitive data type that we rely on heavily when developing applications in some way or another. Often we need to use strings with dynamic values instead of hardcoded ones. For this, we leverage string interpolation that allows us to pass dynamic values in a string.
Now, if we consider the following string, “Color of the item is color.”
We can use string interpolation if we are using this in only one place.
item = 'pen'
color = 'red'
p "Color of the #{item} is #{color}"
# prints
# Color of the pen is red
It won’t work when we need to reuse the template by assigning it into a variable. And duplicating the template everywhere is bad and leads to unmaintainable code.
https://ruby-doc.org/core-2.6/String.html#method-i-25
Enter str % arg, A String class method to substitute values in templates.
I came across this while watching a RailsConf talk on refactoring by Katrina Owen (Highly Recommended).
In order to reuse the template, we can assign it to a variable and pass the values into the “%” method as an Array or Hash.
Array
template = "Color of the %s is %s." # %s - string
p template % ['pen', 'red']
# prints Color of the pen is red.
While using an Array;
- You should specify the placements as %s, %f or %d.
- The order of substitutions should be maintained.
- The change in data type may result in errors.
Hash
Using a Hash is the most convenient and simple way. I can use named placements and never need to think of the list of issues above.
template = "Color of the %{item} is %{color}."
p template % { item: "pen", color: "red" }
# prints Color of the pen is red.